本文最后更新于:1 年前
(一)引言:
在c++中,我们在类中创建成员函数,在创建类的对象后,在类的对象上使用类的成员函数可以访问类的此对象的数据。
那么这个成员函数是怎么知道要访问的是此对象的数据成员,而不是其他对象的呢?
其原因就是每个对象拥有的this指针——每个对象使用成员函数时通过传入自己的this指针来使成员函数能够确定要访问的对象。
下面对this指针进行详细介绍。
(二)概念:
定义:关键字this是指向对象自己的一个常量指针,,所以,this指针是不能够被赋值或者改变的。
this指针是成员函数的隐含形参,换言之,类的对象在正常为成员函数传入参数时,实际上最后还有一个额外的参数位置,参数类型是该类的常量指针,并含有默认值,默认值即为此调用对象的地址,在调用成员函数时,this指针作为实参被传入成员函数。
this指针本质上是成员函数的局部变量。
例子如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| #include <iostream> using namespace std; class Simple { private: int x; int y; public: Simple() = default; Simple (int a, int b) : x(a), y(b) { cout << "Finish the constructed function" << endl; } void resetXY(int a, int b) { x = a,y = b; return ; } ~Simple() { cout << "Finish deconstructor" << endl; } }; int main() { Simple s(1, 2); s.resetXY(2, 3); return 0; }
|
(三)this指针的注意要点:
-
作为常量指针,不能给this指针赋值。
-
this指针的作用域在类成员函数的内部,只能在成员函数内部,在类外无法获取。
-
this指针的创建:
this指针在成员函数的开始执行前构造,并在成员函数的执行结束后清除。
-
只有非静态成员函数才拥有this指针
——友元函数不是类的成员函数,没有this指针。
——静态函数不属于某个对象(详见c++类——静态成员)博客),没有this指针。
-
this指针不可以显式声明,但可以显式使用。
——显式使用是指可以在成员函数内调用this指针,比如使用this地址,(*this).x,this->x
x为数据成员/某些成员函数。
-
this指针不是对象的一部分,其所占的内存大小不会反应在sizeof操作符上。
(四)this指针的显式使用样例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| #include <iostream> using namespace std; class Simple { private: int x; int y; public: Simple() = default; Simple (int a, int b) : x(a), y(b) { cout << "Finish the constructed function" << endl; } void resetXY(int a, int b) { x = a, y = b; return ; } void Simple_thisuse(int a,int b) { cout << this->x << " " << this->y << endl; (*this).x += a; (*this).y += b; cout << this->x << " " << this->y << endl; cout << this << endl; return; } ~Simple() { cout << "Finish deconstructor" << endl; } }; int main() { Simple s(1, 2); s.resetXY(2, 3); s.Simple_thisuse(1, 1); return 0; }
|
结果为: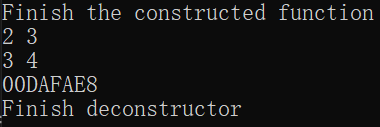